Understanding TypeScript: A Comprehensive Guide for Modern Development
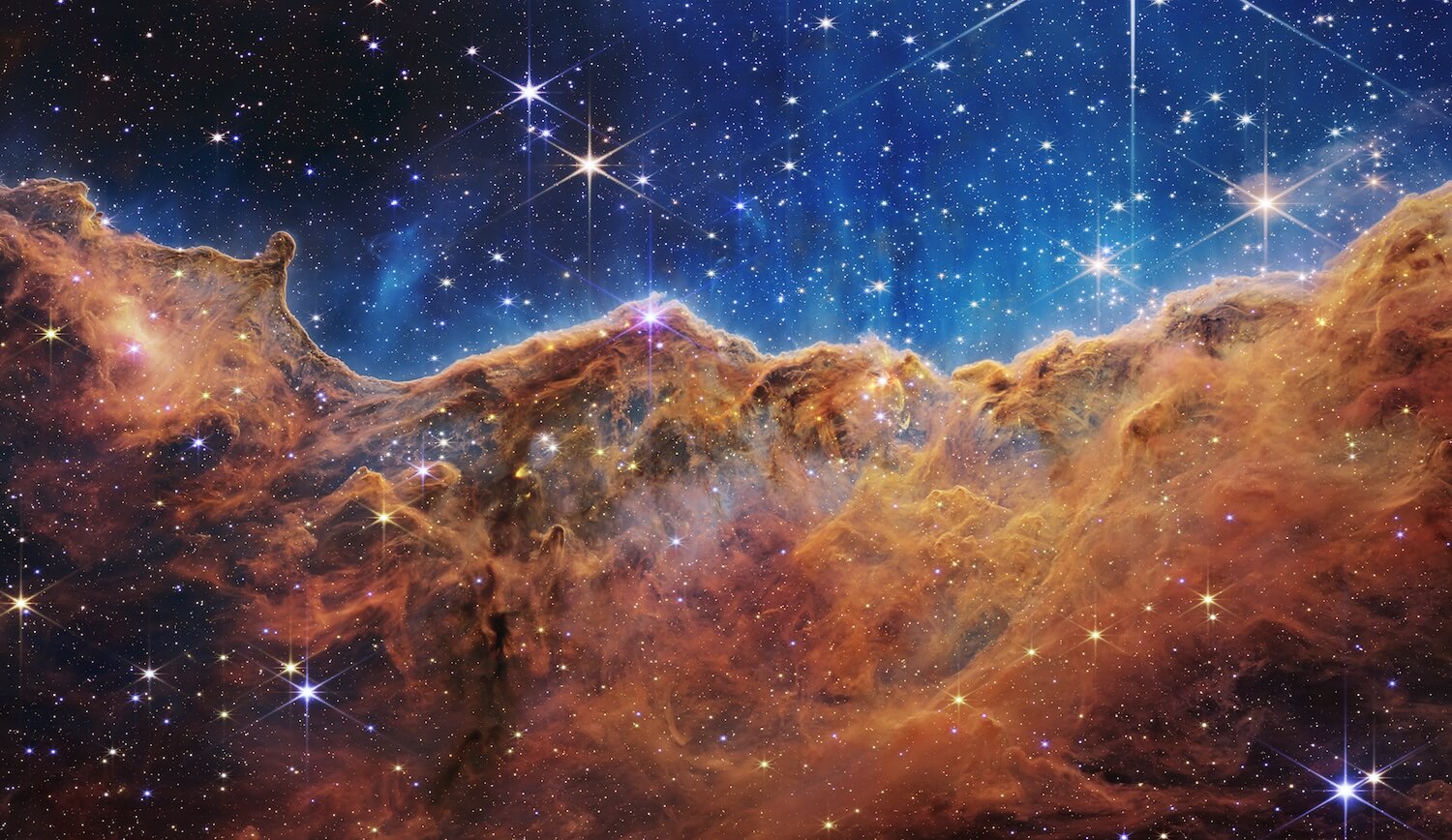
TypeScript has become a cornerstone for modern web and application development, offering robust type safety, improved tooling, and scalability. Its adoption has grown exponentially among developers who value maintainability and error-free coding. This blog post dives deep into TypeScript, its features, benefits, and how it empowers modern development practices.
What is TypeScript?
TypeScript is a superset of JavaScript developed by Microsoft. It extends JavaScript by adding static types, enabling developers to catch errors during development rather than runtime. Despite its additional features, TypeScript compiles down to plain JavaScript, ensuring compatibility with any JavaScript environment.
Why Use TypeScript?
1. Static Typing
TypeScript's static typing is its standout feature. By defining types for variables, functions, and objects, developers can:
- Catch errors early in the development cycle.
- Reduce debugging time.
- Improve code readability and maintainability.
For example:
// JavaScript
function add(a, b) {
return a + b;
}
// TypeScript
function add(a: number, b: number): number {
return a + b;
}
In the TypeScript example, any attempt to pass non-numeric arguments would result in a compile-time error.
2. Enhanced Tooling and IDE Support
TypeScript provides richer IntelliSense, autocomplete, and error-checking features in modern IDEs like VS Code, making coding faster and more efficient.
3. Improved Code Organization
TypeScript supports advanced features like modules, namespaces, and decorators, allowing for better organization in large codebases.
4. Backward Compatibility
TypeScript compiles into clean, ES3-compatible JavaScript, ensuring it works in older browsers while supporting modern syntax.
5. Adoptive Ecosystem
TypeScript seamlessly integrates with popular frameworks and libraries like React, Angular, Node.js, and more.
Key Features of TypeScript
1. Type Annotations
Type annotations specify the data types of variables and function parameters. This prevents unintended type mismatches.
let isActive: boolean = true;
let username: string = "Ebrahim";
2. Interfaces and Types
Interfaces and type
aliases enable the definition of complex types.
type User = {
id: number;
name: string;
email: string;
};
interface Product {
id: number;
name: string;
price: number;
}
3. Generics
Generics provide a way to create reusable components that work with a variety of data types.
function identity<T>(value: T): T {
return value;
}
const num = identity<number>(42); // Works with numbers
const str = identity<string>("TypeScript"); // Works with strings
4. Union and Intersection Types
Union types allow variables to hold multiple types, while intersection types combine types.
type Status = "success" | "error" | "loading";
type Coordinates = { x: number } & { y: number };
5. Enum
Enums define a set of named constants.
enum Role {
Admin,
User,
Guest,
}
const currentUserRole: Role = Role.User;
6. Decorators
TypeScript supports decorators for meta-programming. Commonly used in Angular and NestJS, decorators add functionality to classes, methods, or properties.
function Logger(target: any) {
console.log("Logging:", target);
}
@Logger
class MyClass {}
TypeScript in Modern Development
1. TypeScript with Frontend Frameworks
React: TypeScript enhances React components by providing type safety for props, state, and hooks.
type Props = { title: string; count: number; }; const Counter: React.FC<Props> = ({ title, count }) => ( <h1>{title}: {count}</h1> );
- Angular: Angular is built with TypeScript, leveraging its advanced features like decorators and DI (Dependency Injection).
2. TypeScript with Backend Frameworks
Express: TypeScript improves the robustness of Express applications with type-safe request and response objects.
import express, { Request, Response } from "express"; const app = express(); app.get("/", (req: Request, res: Response) => { res.send("Hello TypeScript!"); });
- Hono: A lightweight framework like Hono gains stronger type safety for building APIs, especially with tools like Drizzle ORM.
3. TypeScript with State Management
TypeScript works seamlessly with state management tools like Zustand and Redux, ensuring predictable application state.
Advanced Concepts
1. Utility Types
TypeScript includes utility types to transform and manipulate types.
Partial<T>
: Makes all properties ofT
optional.Pick<T, K>
: Picks specific properties from a type.Record<K, T>
: Creates a type with keysK
and valuesT
.
2. Mapped Types
Mapped types transform an existing type into a new one.
type ReadonlyType<T> = {
readonly [K in keyof T]: T[K];
};
3. Type Narrowing
TypeScript refines types based on runtime checks.
function logId(id: string | number) {
if (typeof id === "string") {
console.log(`String ID: ${id}`);
} else {
console.log(`Number ID: ${id}`);
}
}
4. Module Resolution
TypeScript supports ES Modules and CommonJS, providing flexibility in project setups.
Best Practices for TypeScript Development
Use
strict
Mode
Always enablestrict
mode intsconfig.json
to enforce stricter type checks.{ "compilerOptions": { "strict": true } }
- Prefer
type
overinterface
Usetype
for flexibility in defining unions and intersections. - Define Narrow Types
Use specific types like"success"
instead ofstring
where applicable. - Avoid
any
Replaceany
with more precise types to maintain type safety. - Leverage Generics
Use generics to create reusable and type-safe code components. - Organize Codebase
Divide code into modules and use barrel files for easier imports.
Setting Up a TypeScript Project
Install TypeScript
npm install -g typescript
Initialize TypeScript Project
tsc --init
Compile TypeScript
tsc
Run TypeScript in Watch Mode
tsc --watch
Conclusion
TypeScript has transformed how developers write JavaScript by adding powerful type-checking and modern features. Whether building frontend applications, backend services, or complex full-stack systems, TypeScript provides unparalleled reliability and scalability. Adopting TypeScript not only boosts productivity but also ensures code quality and future-proofing in an ever-evolving tech landscape.
So why wait? Start your TypeScript journey today and elevate your development practices!