Understanding Object-Oriented Programming: A Beginner's Guide
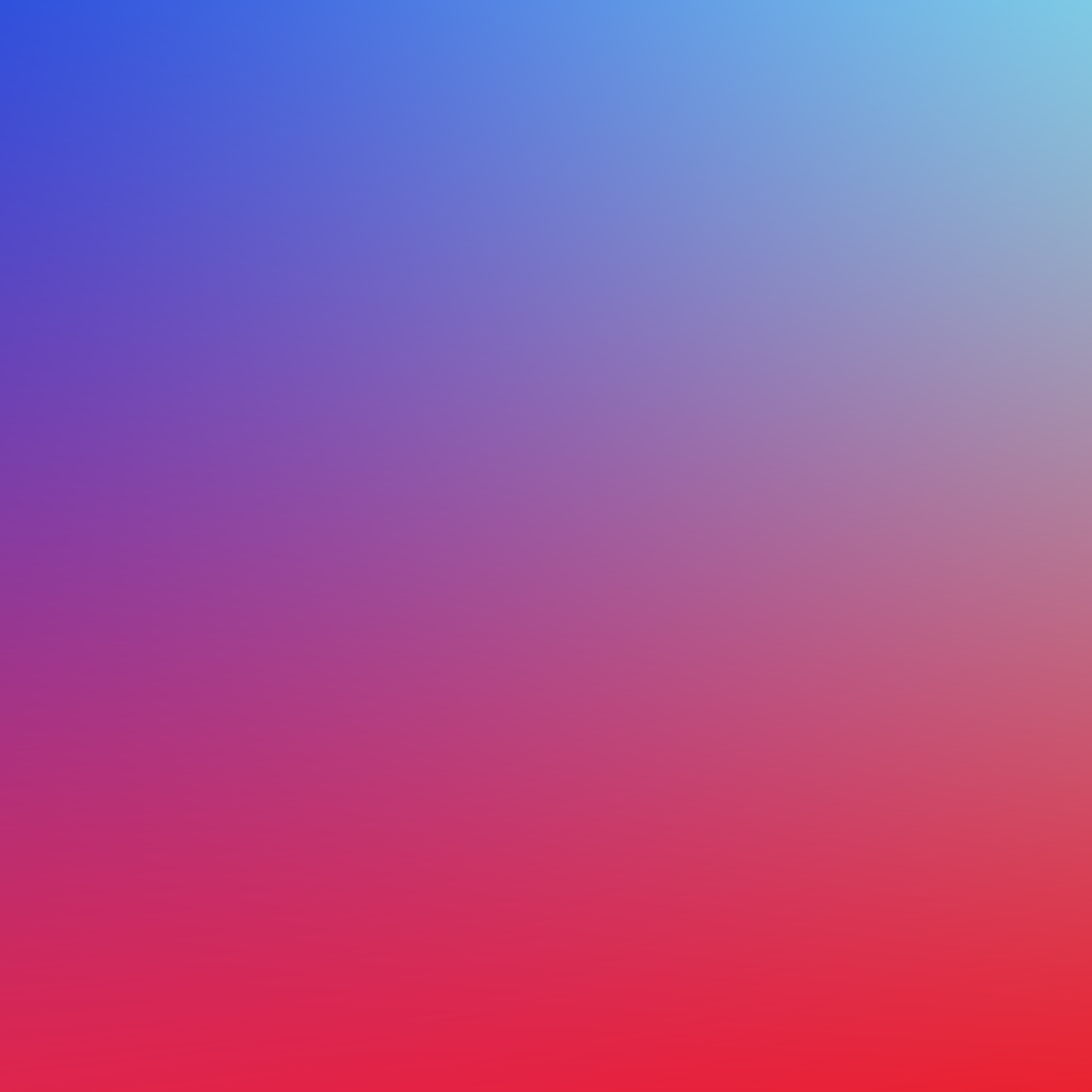
Object-Oriented Programming (OOP) is a programming paradigm that has revolutionized how we write and organize code. Let's dive into its core concepts and understand why it's so widely used in modern software development.
What is Object-Oriented Programming?
OOP is a programming paradigm based on the concept of "objects" that contain both data and code. These objects can interact with one another and can be used to model real-world entities in our programs.
The Four Pillars of OOP
1. Encapsulation
Encapsulation is the bundling of data and the methods that operate on that data within a single unit or object. It restricts direct access to some of an object's components, preventing unintended interference and misuse.
class BankAccount:
def __init__(self):
self.__balance = 0 # Private variable
def deposit(self, amount):
if amount > 0:
self.__balance += amount
def get_balance(self):
return self.__balance
2. Inheritance
Inheritance allows classes to inherit attributes and methods from other classes. This promotes code reuse and establishes a relationship between parent and child classes.
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
pass
class Dog(Animal):
def speak(self):
return f"{self.name} says Woof!"
3. Polymorphism
Polymorphism allows objects to take multiple forms. The most common use of polymorphism is when a parent class reference is used to refer to a child class object.
class Animal:
def speak(self):
pass
class Dog(Animal):
def speak(self):
return "Woof!"
class Cat(Animal):
def speak(self):
return "Meow!"
# Polymorphic behavior
def make_animal_speak(animal):
print(animal.speak())
# Same function works with different animal types
dog = Dog()
cat = Cat()
make_animal_speak(dog) # Output: Woof!
make_animal_speak(cat) # Output: Meow!
In this example, make_animal_speak() demonstrates polymorphism by working with any object that inherits from Animal and implements the speak() method. The function doesn't need to know the specific type of animal - it just knows that the object will have a speak() method.
4. Abstraction
Abstraction means hiding complex implementation details and showing only the necessary features of an object. This helps in managing complexity in large software systems.
class Shape:
def __init__(self, name):
self.name = name
def calculate_area(self):
pass # Abstract method
class Rectangle(Shape):
def __init__(self, name, width, height):
super().__init__(name)
self.width = width
self.height = height
def calculate_area(self):
return self.width * self.height
# Using abstraction
shape = Rectangle("Rectangle1", 5, 3)
print(f"Area of {shape.name}: {shape.calculate_area()}") # Output: Area of Rectangle1: 15
In this example, the Shape class provides an abstract method calculate_area(), while the Rectangle class implements the specific calculation. Users of Rectangle only need to know how to call calculate_area(), not the implementation details.
Benefits of OOP
- Modular development of code
- Code reusability
- Flexibility through polymorphism
- Data hiding and abstraction
- Easier maintenance and modification
Conclusion
Object-Oriented Programming is more than just a programming concept—it's a distinct way of thinking about software design. By understanding and applying these principles, you can create more organized, maintainable, and scalable code.
From small projects to large-scale applications, OOP principles help you build better software solutions.