Mastering Clean Code: Best Practices for Better Software Development
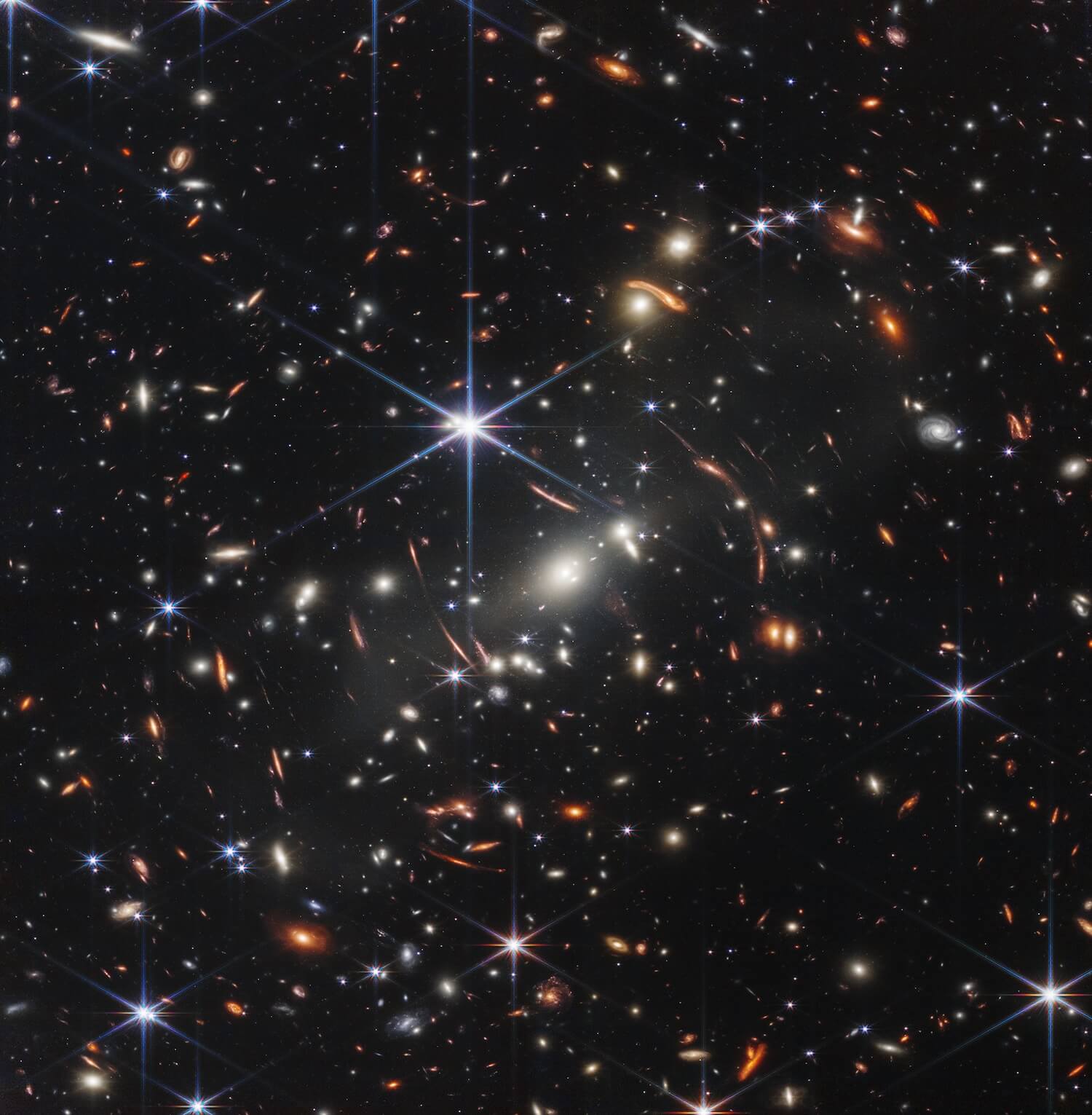
In today's fast-paced software development world, writing clean code isn't just a luxury—it's a necessity. Let's explore some essential practices that can help you write more maintainable and efficient code.
1. Meaningful Names Matter
One of the fundamental aspects of clean code is using clear, descriptive names for variables, functions, and classes. Consider these examples:
// Bad
const d = new Date();
const x = users.filter(u => u.a > 18);
// Good
const currentDate = new Date();
const adultUsers = users.filter(user => user.age > 18);
2. Keep Functions Small and Focused
Functions should do one thing and do it well. This principle, known as the Single Responsibility Principle, makes code easier to test, maintain, and understand.
3. Comments: Use Them Wisely
Good code should be self-documenting. Comments should explain why something is done, not what is being done. The code itself should be clear enough to explain the what.
# Bad
# Increment x by 1
x += 1
# Good
# Increment counter to account for new user registration
user_count += 1
4. Consistent Formatting
Maintaining consistent formatting throughout your codebase makes it more readable and professional. Use automated formatting tools when possible.
5. Error Handling
Proper error handling is crucial for robust applications. Don't just catch exceptions—handle them meaningfully:
try {
processUserData(userData);
} catch (InvalidDataException e) {
logger.error("Invalid user data format: " + e.getMessage());
notifyUser("Please check your input and try again");
}
Conclusion
Clean code isn't about following rules blindly—it's about making your code more maintainable, readable, and efficient. Start implementing these practices in your next project, and you'll see the benefits in the long run.
Remember: Code is read much more often than it is written. Make it count!